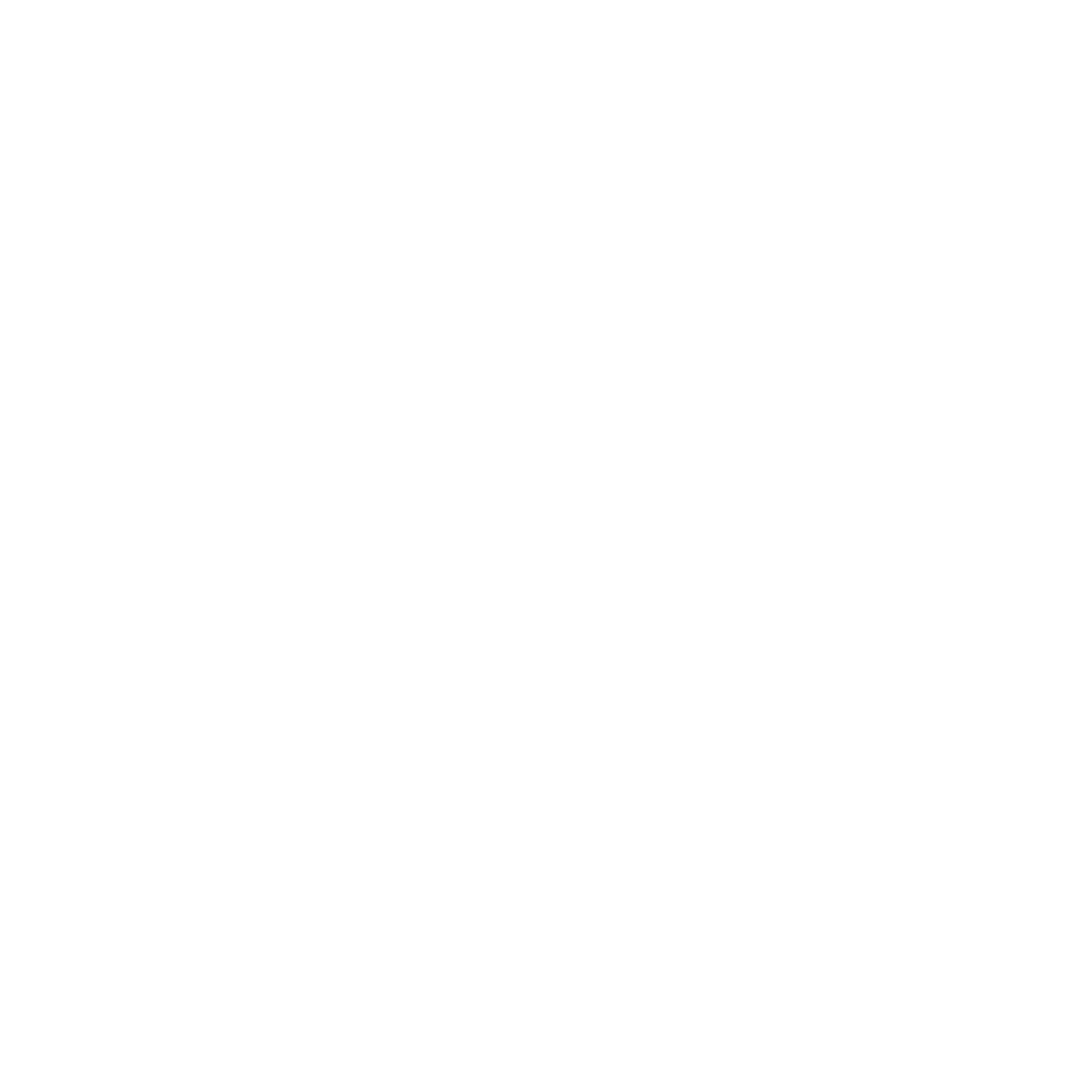
Integrating Angular with ASP.NET Core RESTful Services (Pluralsight)
Course Description
Learn how to build an Angular and ASP.NET Core application that can perform create, read, update and delete (CRUD) operations. The "Integrating Angular with ASP.NET Core RESTful Services" course starts off by demonstrating how to get started creating the project structure so that you understand the different technologies and how they will interact with each other. It then shows how to build an ASP.NET Core RESTful service to expose GET, POST, PUT and DELETE actions to clients. The RESTful service actions are then consumed by an Angular application using a reusable service class, the built-in Http client and RxJS functionality such as observables. By the end of the course you'll understand the process involved to not only create RESTful services using ASP.NET Core, but also how Angular can be used to consume these services and display and capture data in the user interface.
View this course at http://www.pluralsight.com
Key Angular Topics Covered
- TypeScript and how it can be used in Angular applications
- Code organization with Angular modules
- The role of ES2015 module loaders in Angular applications
- Promises versus Observables
- Learn how Observables work (a nice visual explanation is shown) and how to subscribe to them
- Learn how to create and use Angular services
- Angular’s Http service and how it can be used to call into RESTful services. The course application also includes code to show how to use the new HttpClient as well if you’re on Angular 4.3+.
- Differences between Template-driven and Reactive forms in Angular
- Directives used in Template-driven forms and how to use them for two-way data binding
- Directives and code used in Reactive forms
- Form validation techniques and custom validators
- How to build custom components and leverage Input and Output properties
- Working with headers sent by the server
- Building a custom pagination component
- CSRF attacks and how Angular can help
Key ASP.NET Core Topics Covered
- Understand GET, POST, PUT and DELETE and the role each plays with RESTful services
- Use ASP.NET Core middleware
- Create RESTful services capable of supporting CRUD operations using C# and ASP.NET Core
- Use Entity Framework Core for data access
- Paging data
- Working with headers on the server-side and client-side
- Preventing CSRF attacks
Prerequisites
To get the most out of this course you should already know the following:
- JavaScript and TypeScript
- Angular v2 or higher fundamentals
- C# fundamentals
- ASP.NET Core fundamentals
Audience
Course Outline
- Course Introduction
- Pre-requisites to Maximize Learning
- Learning Goals
- Server-side Technologies and Concepts
- Client-side Technologies and Concepts
- Running the Application on Windows
- Running the Application on Mac
- Running the Application with Docker
- Exploring the Angular and ASP.NET Core Application
- Getting Started – Using a Seed Project
- Getting Started – Using the dotnet CLI
- Getting Started – Using the Angular CLI
- Exploring the Project Structure
- Application Packages and Modules
- Configuring the ES Module Loader
- Angular Modules, Components and Services
- Retrieving Data Using a GET Action
- Injecting Data Repository and Logging Functionality
- Creating a GET Action to Return Multiple Customers
- Creating a GET Action to Return States
- Creating a GET Action to Return a Single Customer
- Making GET Requests with an Angular Service
- Subscribing to an Observable in a Component
- Displaying Customers in a Grid
- Displaying a Customer in a Form
- Converting to a Reactive Form
- Inserting Data Using a POST Action
- Creating a POST Action to Insert a Customer
- Making a POST Request with an Angular Service
- Modifying the Customer Form to Support Inserts
- Exploring the Reactive Form
- Modifying the Reactive Form submit() Function
- Updating Data Using a PUT Action
- Creating a PUT Action to Update a Customer
- Making a PUT Request with an Angular Service
- Modifying the Customer Form to Support Updates
- Exploring the Reactive Form
- Viewing Swagger Documentation
- Deleting Data Using a DELETE Action
- Creating a DELETE Action to Delete a Customer
- Making a DELETE Request with an Angular Service
- Modifying the Customer Form to Support Deletes
- Exploring the Reactive Form
- Viewing Swagger Documentation
- Data Paging, HTTP Headers and CSRF
- Adding a Paging Header to a RESTful Service Response
- Accessing Headers and Data in an Angular Service
- Adding Paging Support to a Component
- Adding a Paging Component
- CSRF Overview
- Adding CSRF Functionality
- Using a CSRF Token in an Angular Service