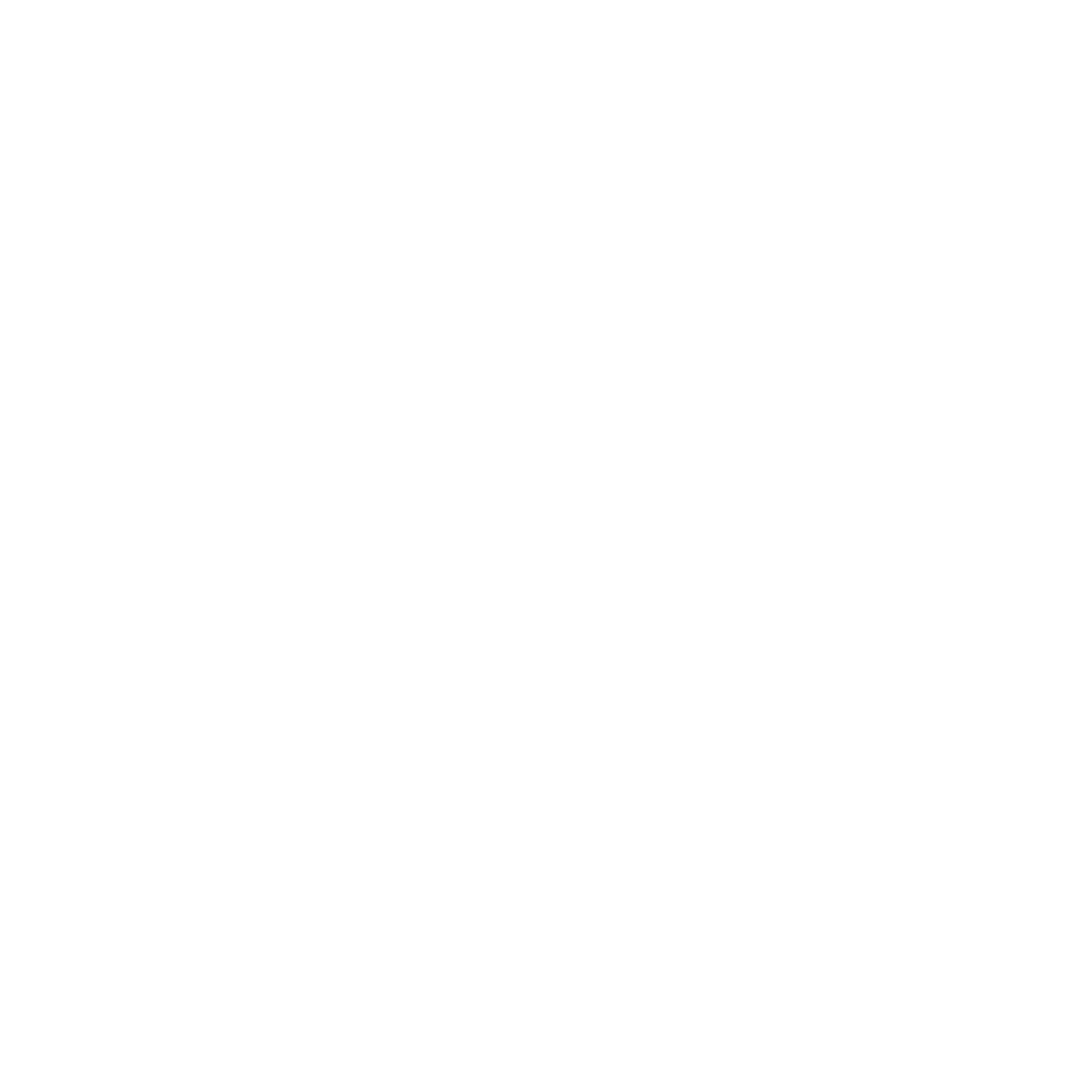
C# Programming with Visual Studio
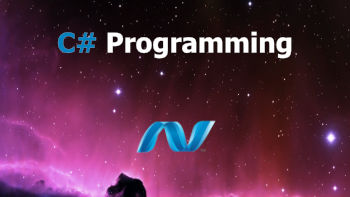
Course Length: 4 Days
Course Description
The C# Programming with Visual Studio course provides developers with the skills and knowledge they'll need to succeed by providing an in-depth and hands-on analysis of different parts of the C# language. Techniques for maximizing productivity using Visual Studio are also covered such as code snippets, debugging techniques, visual database queries, and more.
The course begins by providing a look at the different components of the .NET Framework and .NET Core and discusses the basic building blocks of the C# language including variables, conditionals and looping. Next, Object-oriented features found in C# are discussed so that students understand how to leverage concepts such as inheritance, method overriding, interfaces, and object hierarchies. The course also shows several of the exciting language features available in C# including lambda expressions and LINQ to Objects. Other topics covered include and introductory look at data access technologies.
This course is taught by Dan Wahlin (a member of the Microsoft MVP and Regional Directors groups).
Prerequisites
This course is designed for software developers with previous programming experience using a programming language such as Visual Basic, C++, Java or other languages.
Audience
Course Outline
- Getting Started with .NET
- What is the .NET Framework and .NET Core?
- The role of the Common Language Runtime (CLR)
- .NET Compilation Model
- Assemblies and Namespaces Overview
- Language Syntax
- C# Fundamentals
- Defining Variables, Constants and Arrays
- Type Casting
- Conditionals and Looping
- Building Classes
- What is a Class?
- Creating and Instantiating Classes
- Defining Fields and Properties
- Defining Methods
- Defining Constructors
- Events and Event Handlers
- The Role of Namespaces
- Object-Oriented Programming
- What is Object-Oriented Programming (OOP)?
- Object-Oriented Programming Concepts
- Method Overloading
- Method Overriding
- Exception Handling
- Generics
- Why do we need Generics?
- Templates and Generics
- The default Keyword and Nullable Types
- System.Collections.Generic Namespace
- Interfaces
- What is an Interface?
- Creating Interfaces
- Implementing Interfaces
- Leveraging .NET Framework Interfaces
- Base Class Library
- The Base Class Library (BCL)
- Working with Dates and Times
- Working with Strings
- Working with Threads
- Using Language Integrated Query (LINQ)
- What is LINQ?
- Extension Methods
- Lambda Expressions
- Using LINQ
- Data Access Technologies
- Data Access Options
- ADO.NET Providers and Classes
- Using DataAdapter and DataSet
- Entity Framework