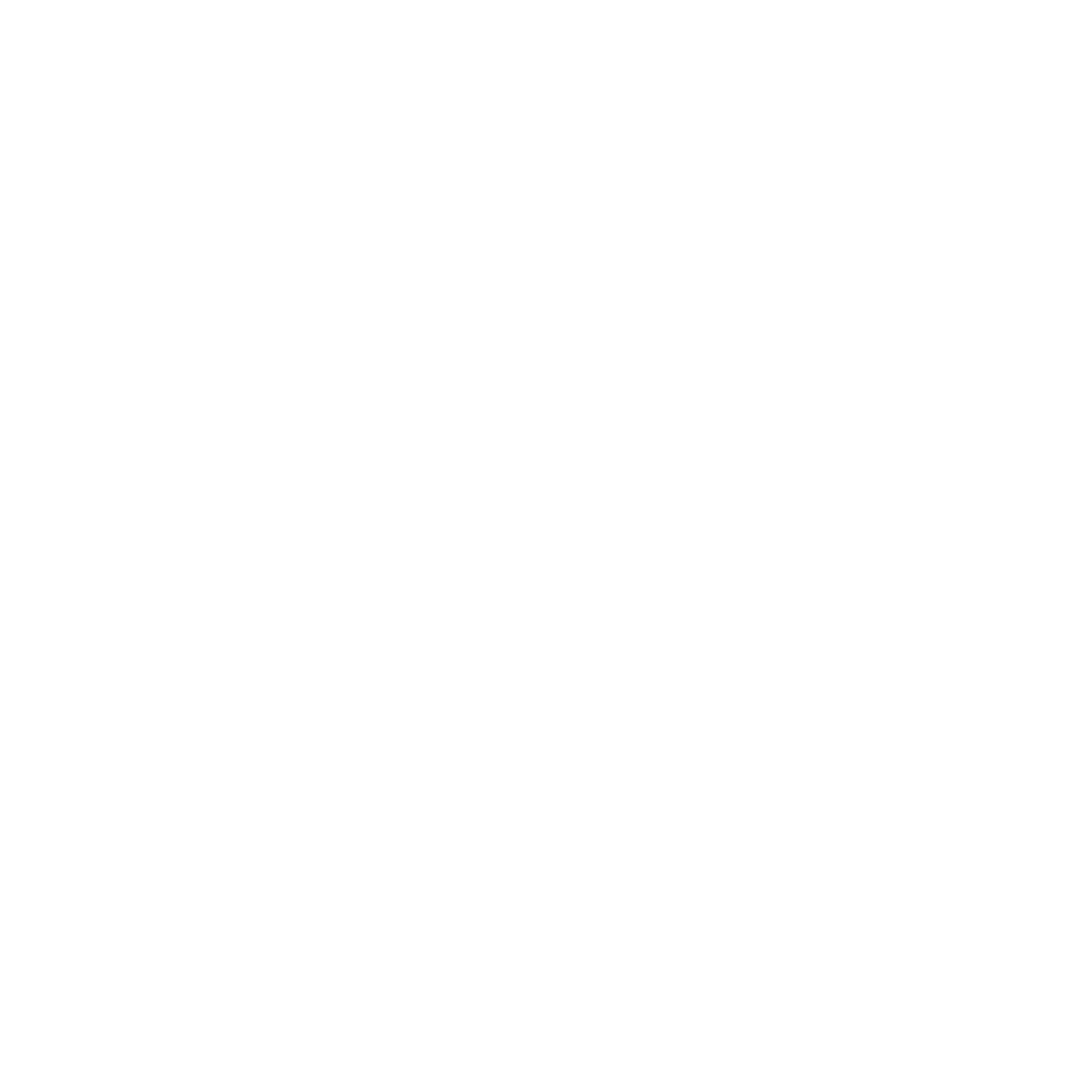
Angular and TypeScript Single Page Application (SPA) Development
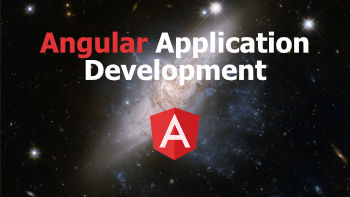
Course Length: 4 Days
Course Description
Angular is the new version of Google’s popular Single Page Application (SPA) framework that can be used to build robust, client-centric applications. With enhanced speed, the ability to leverage the latest language features and a more modular architecture, Angular offers a cutting-edge approach to building today’s applications.
“Learn by doing!” – that’s the goal of the Angular Application Development course. The course starts with an introduction to Single Page Applications (SPA) and the benefits that SPAs can provide to both developers and end users. It then jumps into an overview of key Angular features and ES2015/TypeScript. Students will learn what benefits ES2015 and TypeScript offer and get hands-on experience using them throughout the class. From there students learn about different aspects of the Angular framework such as components, modules, templates, data binding syntax, directives, data services, calling RESTful services, observables, decorators, routing and more. Best practices and techniques for structuring code are discussed as well as techniques for using the documentation and Angular Github source code repository to find answers to questions.
This course is taught by Google Developer Expert (GDE) Dan Wahlin.
Note: This course covers the latest version of Angular. It doesn't cover the AngularJS 1.x version of the framework. We offer a separate AngularJS course as well.
Prerequisites
Attendees must have prior experience working with JavaScript, HTML and CSS to take this class. A minimum of 6-months of hands-on JavaScript (ES5 and ES2015) experience is recommended to get the most out of the course.
Experience working with TypeScript is also highly recommended since TypeScript and ES2015 will be used throughout the course.
For development teams that are new to ES2015 or TypeScript we offer a 1-day TypeScript workshop that can be run the same week as the Angular course. We highly recommend adding this day if your team doesn’t have any experience with ES2015 or TypeScript.
Audience
Course Outline
- Introduction to Angular
- Angular Building Blocks
- Angular Feature Overview
- The Big Picture
- Angular CLI
- Angular Application Flow
- Angular Docs
- AngularJS to Angular
- The Angular JumpStart Application
- Application Overview
- Angular JumpStart in Action
- Application Structure
- Components and Modules
- Components Overview
- Component Lifecycle
- ES2016 Modules
- Angular Modules
- Template Expressions and Pipes
- Templates Syntax Overview
- Interpolation and Expressions
- Working with Pipes
- Component Properties and Data Binding
- Property and Event Binding
- Input and Output Properties
- Angular Directives
- Two-way Binding
- Change Detection
- Services, Providers and HttpClient
- Services Overview
- Injectors and Providers
- @Injectable Decorator
- Promises and Observables
- Calling RESTful Services with HttpClient
- Routing
- Routing Overview
- Routing Steps
- The Router Service
- Route Parameters
- Creating Child Routes
- Route Guards and Lazy Loading
- Introduction to Route Guards
- Creating and Using Route Guards
- Lazy Loading
- Forms
- Forms Overview
- Template-Driven Forms
- Reactive Forms
- Managing Form Control Styles
- TypeScript JumpStart (Bonus)
- Introduction to TypeScript
- Types, Keywords and Hierarchy
- Classes, Properties and Functions
- Interface Constraints
- Namespaces and Modules
- Compiling TypeScript
- Bonus - Custom Directives and Components
- Directives Overview (Attribute vs. Structural)
- Building a Custom Sorting Directive
- Building a Custom FilterTextbox Component
- Building a Custom Mapping Component
- Bonus - Webpack Fundamentals
- Webpack Overview
- Webpack Building Blocks
- Ahead of Time Compilation in Angular
- Bonus - Unit Testing
- Angular Unit Testing Features
- Unit Testing Players
- Test Suites, Specs and Expectations Overview
- Creating an Angular Service Test Suite and Spec
- Creating an Angular Component Test Suite and Spec
- Mocking Objects