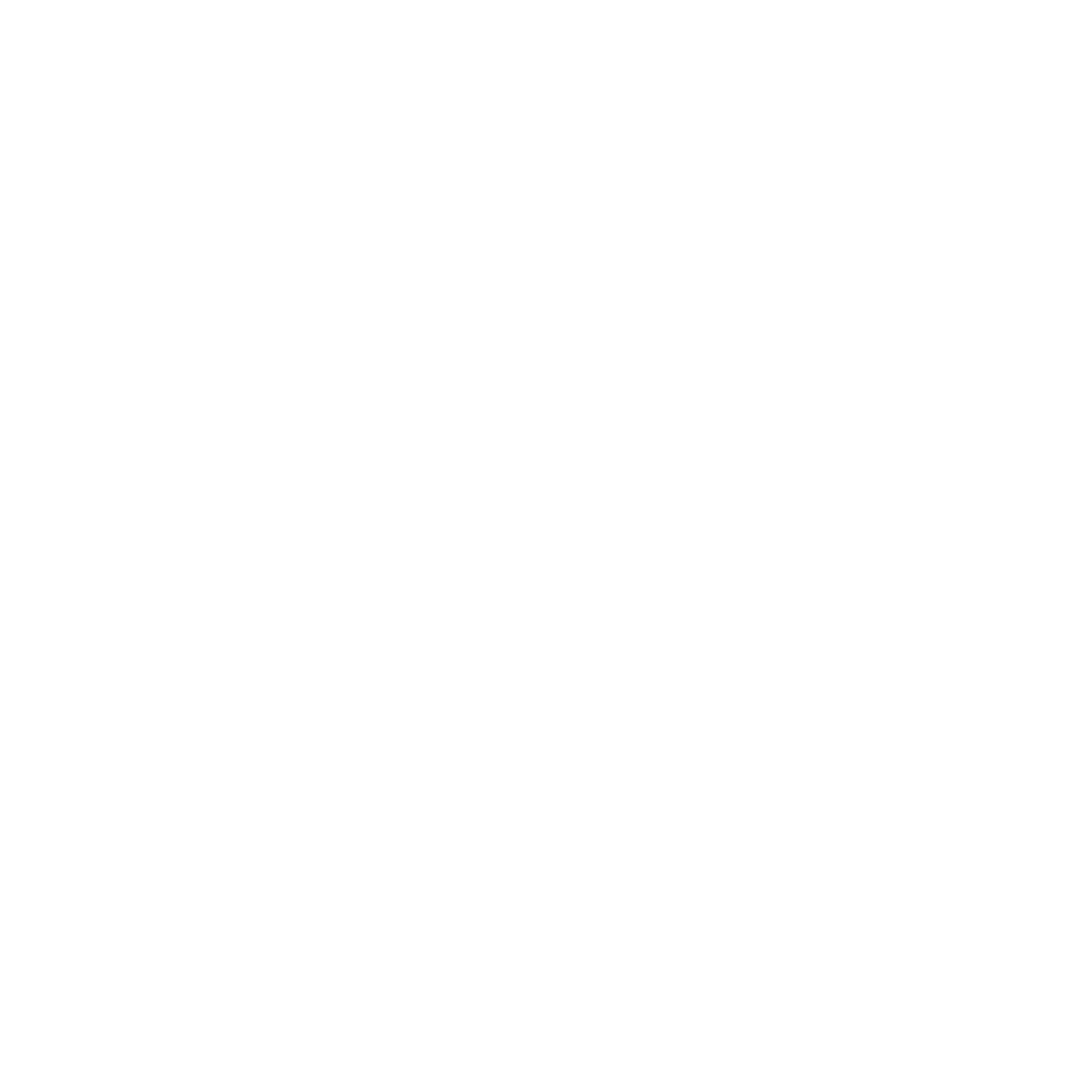
Angular Architecture and Best Practices (Pluralsight)
Course Description
There are a lot of questions out there about the core concepts of Angular, such as whether or not you’re following established best practices, how easy will it be to maintain and refactor the application in the future, how do I structure my features, modules, components, services, and more? Whether you’re starting a new application from scratch or updating an application, what application architecture should be used?
In the Angular Architecture and Best Practices course you’ll learn about different architectural concepts, best practices, and techniques that can be used to solve some of the more challenging tasks that come up during the planning and development process. You’ll learn about a planning template that can be used to provide a simple and efficient way to get started. Discover different component communication techniques, walk through state management and code organization options. Finally, you’ll explore general best practices, performance considerations, and much, much more.
When you’re finished with this course, you’ll have the skills and knowledge needed to think through the process of building a solid application that is easy to refactor and maintain.
View this course at http://www.pluralsight.com.
Here are a few questions this course will help you answer:
- Is there one “right” way to architect and build an Angular application? Short answer – NO!
- What are some key concepts I should consider when planning my application architecture?
- Is there any type of planning template I can use to help my team get started?
- Is it important to think through the organization of modules or should I put everything in the root module?
- What’s the difference between shared and core modules?
- How do I structure components? What if I have deeply nested components?
- How should I organize my application features?
- How do I communicate between components? What if I need to communicate across multiple levels of an application?
- What best practices should I be following throughout my application?
- Do I need a state management solution? What are some of the available options and how do they compare?
- What is an observable service and how would I create and use one?
- Can reference types and value types have different affects on my application behavior?
- How do I share code in my application? What if I need to share code between multiple applications?
- What’s an RxJS subject? Is there more than one type of subject?
- How can I use forkJoin, concatMap, switchMap, mergeMap, and other RxJS operators to make more efficient calls to the server?
- Where should I consider using HTTP Interceptors in my app?
- Is it OK to call component functions from a template? Are there alternatives I should consider?
- What different techniques can be used to unsubscribe from observables?
- What are some key security considerations I should be thinking about?
- And much more…
Prerequisites
Existing knowledge of Angular and TypeScript is required.
Audience
Course Outline
- Course Introduction
- Planning the Application Architecture
- Introduction
- Architecture Considerations
- Architecture Planning Template
- Architecture Planning Template Example
- The Angular Style Guide
- Other Considerations
- Organizing Features and Modules
- Organizing Features
- Feature Modules
- Core and Shared Modules
- Core and Shared in Action
- Creating a Custom Library
- Consuming a Custom Library
- Putting All the Modules Together
- Structuring Components
- Container and Presentation Components
- Container and Presentation Components in Action
- Passing State with Input and Output Properties
- Input and Output Properties in Action
- Change Detection Strategies
- Reference vs. Value Types
- Cloning Techniques
- Cloning in Action
- Cloning with Immutable.js
- Component Inheritance
- Component Inheritance in Action
- Component Communication
- Component Communication Techniques
- Understanding RxJS Subjects
- RxJS Subject in Action - Part 1
- RxJS Subject in Action - Part 2
- Creating an Event Bus Service
- Using an Event Bus Service
- Creating an Observable Service
- Using an Observable Service
- Unsubscribing from Observables
- State Management
- The Need for State Management
- State Management Options
- Angular Services
- Ngrx
- Ngrx in Action
- ngrx-data
- ngrx-data in Action
- Observable Store
- Observable Store in Action
- State Management Review
- Additional Considerations
- Functions versus Pipes
- Functions and Pipes in Action
- Using a Memo Decorator
- HttpClient and RxJS Operators
- Key Security Considerations
- HTTP Interceptors
- Course Summary